Android - The difference between layout_gravity and gravity
- Dan Thompson
- Feb 13, 2018
- 1 min read
In short: layout_gravity moves the whole View, gravity moves the content inside the view.
The best way I can think to explain this concept is to show two different ways to center some content on the screen.
The first way uses the layout_gravity attribute:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:orientation="vertical"
tools:context="com.example.android.courtcounter.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Team A"
android:layout_gravity="center"
android:layout_margin="10dp" />
</LinearLayout>
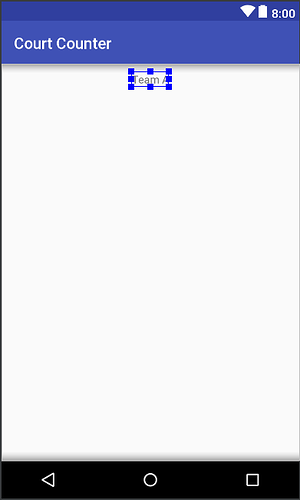
The main things to note about this example is that the TextView has a line android:layout_width =“wrap_content”. This means that the box surrounding the text view (seen in blue on the screenshot) is tight to the content inside it. The line: android:layout_gravity=“center” is therefore moving the whole TextView into the center of the parent, LinearLayout.
The second way uses the gravity attribute:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" tools:context="com.example.android.courtcounter.MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Team A" android:gravity="center"
android:layout_margin="10dp" />
</LinearLayout>
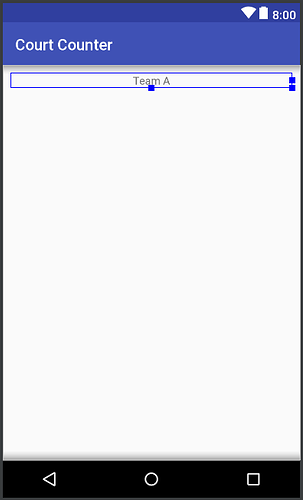
There have only been two changes to the code from the earlier example. Firstly, the TextView now has a line android:layout_width =“match_parent”. This means that the box surrounding the text view (seen in blue on the screenshot) is stretched across the whole screen. The line: android:gravity=“center” is therefore moving content of the TextView (i.e. “Team A”) into the center of its expanded view.
Comentarios