Android - Creating Intents: Contacts/People
- Dan Thompson
- Feb 10, 2018
- 4 min read
Hello all,
I really enjoyed the Udacity Android Basics course by Google lesson Practice with Intents so I am taking some time to fully explore each of the intents in the Android Common Intents documentation. The one I have most recently looked at is Contacts/People App.
First I just created a simple button to activate the intent and an empty TextView to populate with a retrieved phone number. The app looks like this at first:
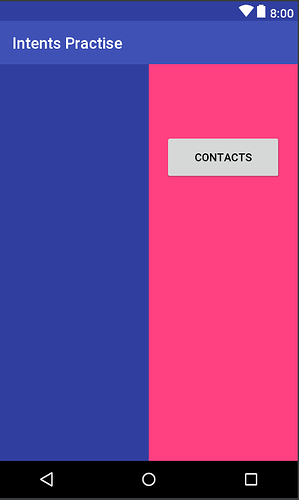
And the code in activity_main.xml looks like this:
<Button
android:id="@+id/get_contact"
android:layout_width="@dimen/button_width"
android:layout_height="@dimen/button_height"
android:layout_gravity="center"
android:layout_margin="@dimen/margin"
android:onClick="getContact"
android:text="Contacts"/>
<TextView
android:id="@+id/contact_number"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
The next thing you need is to get permission to read something in your contacts app. To do this you need to go to Apps > Manifest > AndroidManifest.xml…
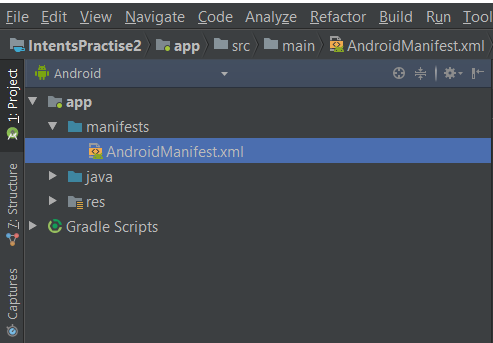
The code originally looks like this:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.android.intentspractise">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
You need to add this extra line of code INSIDE the section but OUTSIDE all other sections of this file. That new line looks like this:
<uses-permission android:name="com.android.alarm.permission.READ_CONTACTS" />
The updated file should therefore look like this, with comments:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.android.intentspractise">
<!--The line below has been changed to give this app permission to take information from the Contacts app
N.B. The permission line HAS to be put here, inside the manifest section but outside the other
sections -->
<uses-permission android:name="com.android.alarm.permission.READ_CONTACTS" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Then I created an activity in MainActivity.java to call the intent when the button is clicked. To launch a contacts/people app all you need to do is include this code.
static final int REQUEST_SELECT_PHONE_NUMBER = 2;
public void getContact(View view) {
// Start an activity for the user to pick a phone number from contacts
Intent intent = new Intent(Intent.ACTION_PICK);
intent.setType(ContactsContract.CommonDataKinds.Phone.CONTENT_TYPE);
if (intent.resolveActivity(getPackageManager()) != null) {
startActivityForResult(intent, REQUEST_SELECT_PHONE_NUMBER);
}
}
Notice that the line: static final int REQUEST_SELECT_PHONE_NUMBER = 2; is added above the intent and that this variable is then used in this line startActivityForResult(intent, REQUEST_SELECT_PHONE_NUMBER); at the end of the intent. This variable is important when doing startActivityForResult because with these intents something is expected to be returned to your app and it needs a unique id number. Because it can only be a number it is helpful to turn it into a static variable so you can give it a more meaningful name.
In order to access and use data from your Contacts app (in this example it is a phone number but it could be any data from Contacts), you need to write another block of code, shown below with comments:
/**
* This method uses the data sent from contacts and sends it to a TextView
* @param requestCode checks that REQUEST_SELECT_PHONE_NUMBER has been returned
* @param resultCode checks that the User did select some information
* (here a phone number) to be returned
* @param data is the phone number itself
*/
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_SELECT_PHONE_NUMBER && resultCode == RESULT_OK) {
// Get the URI and query the content provider for the phone number
Uri contactUri = data.getData(); String[] projection = new String[]{ContactsContract.CommonDataKinds.Phone.NUMBER}; Cursor cursor = getContentResolver().query(contactUri, projection, null, null, null);
// If the cursor returned is valid, get the phone number
if (cursor != null && cursor.moveToFirst()) {
int numberIndex = cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER);
String number = cursor.getString(numberIndex);
// Send the phone number to the TextView with id contact_number
contact_number.setText(number);
}
}
}
You need to do two more things before the phone number can be seen on your app screen. Add a line of code to the onCreate method and add a new Global variable. This will make the top of your Java code look like this:
private TextView contact_number;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
contact_number = (TextView) findViewById(R.id.contact_number);
}
The key changes that have been made here are the additions of the lines contact_number = (TextView) findViewById(R.id.contact_number); inside the onCreate method and this line private TextView contact_number; outside of the onCreate method. These two lines get the empty TextView contact_number from the the activity_main.xml file then turns it into a global variable so that the onActivityResult method can use it to put he photo in.
All of this should allow you to use the Contacts/People intent in your app and save a telephone number from it on your app screen. At the end your screen should look something like this:
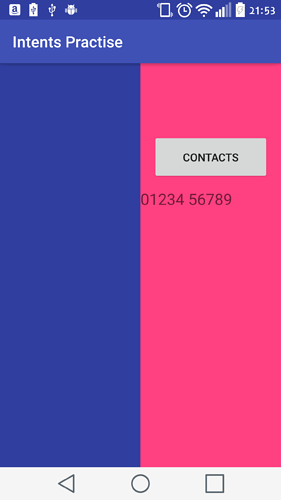
This article is only really scratching the surface of what you can do with the Contacts/People App. You can retrieve and display other types of information from your Contacts/People App as well. You can also edit contacts from in your App and Add new contacts. There is also much more you can do.
Please feel free to question, correct or further explain anything I have covered here. I am by no means an expert on this topic and would love anything that will help me learn more.
Links:
Recent Posts
See AllI find that adding little notes to myself in my code is very helpful sometimes. It means that I can write pseudocode directly into...
Comments