Android - Creating Intents: Local Actions (Call a Taxi)
- Dan Thompson
- Feb 8, 2018
- 3 min read
Hello all,
I really enjoyed the Udacity Android Basics Course by Google lesson Practice with Intents so I am taking some time to fully explore each of the intents in the Android Common Intents documentation. The one I have most recently looked at is Local Action (Call a Taxi).
First I just created a simple button to activate the intent. The app looks like this at first:
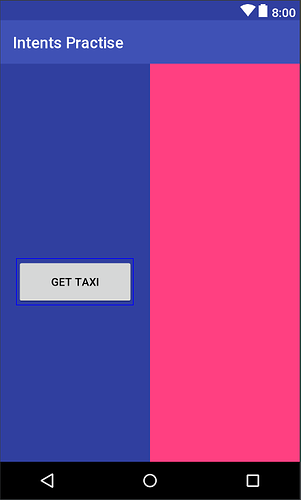
And the code in activity_main.xml looks like this:
<Button
android:id="@+id/taxi"
android:layout_width="@dimen/button_width"
android:layout_height="@dimen/button_height"
android:layout_gravity="center"
android:layout_margin="@dimen/margin"
android:onClick="getTaxi"
android:text="Get Taxi"/>
Then I created an activity in MainActivity.java to call the intent when the button is clicked.
public void getTaxi(View view) {
Intent intent = new Intent(ReserveIntents.ACTION_RESERVE_TAXI_RESERVATION);
if (intent.resolveActivity(getPackageManager()) != null) {
startActivity(intent);
} else {
Toast.makeText(this, "No App Available", Toast.LENGTH_SHORT).show();
}
}
If you do this you will find at first that the words “ReserveIntents” is highlighted in red. To fix this problem what you need to do is add a line to the build.gradle file. Now, this is NOT the one to be found in or underneath the gradle folder! It is actually found in the app folder below the src folder. See below image…
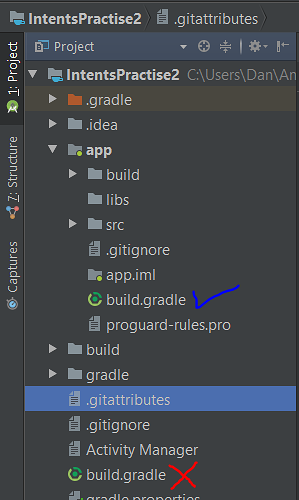
Thanks to my incredibly well designed annotations you should see that you need to pick the file marked with the blue tick, not the one with a red cross. Clicking on this will take you to the file you can see below…
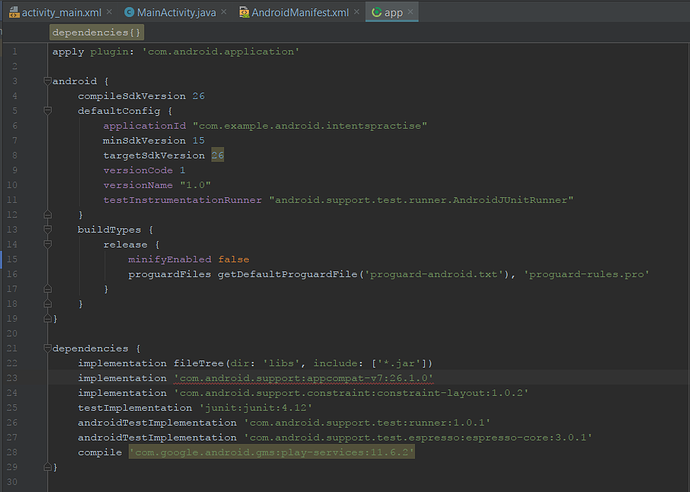
Your file should look similar to this one. Don’t worry if some lines are different. The line we need to add is the one highlighted in yellow: compile 'com.google.android.gms:play-services:11.6.2'. This version of play-services should work for now but as it is updated you will need to change this version number: 11.6.2 to whatever the latest version of google play services your version of Android Studio is running. Then you need to go back to your MainActivity.java file and near the top click on the + sign next to “import …” to open up all the imports for your file.
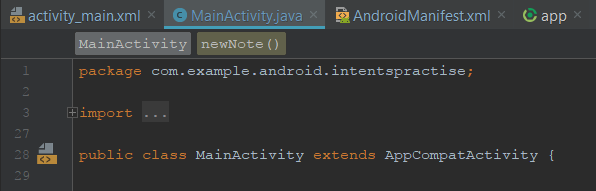
That should open up a list of files imported to your app that looks something like this:
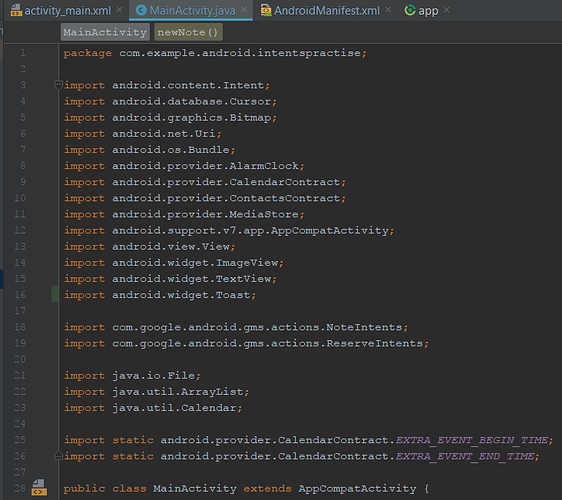
Again, don’t worry if your list is slightly different to the one here. The only line you need to add is: import com.google.android.gms.actions.ReserveIntents;
What you also may need to do is install Google Play Services. To do that follow these steps:
Go to Tools > Android > SDK Manager, as seen in the image below) and click on it.
Then, go to Appearance and Behaviour > System Settings > Android SDK; click the SDK Tools tab and ensure that the “Google Play Services” checkbox is ticked. Then click “Apply” and wait for it to download.

The final thing you need is to get permission to call a taxi. To do this you need to go to Apps > Manifest > AndroidManifest.xml…

The code originally looks like this:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.android.intentspractise">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
You need to add this extra line of code INSIDE the section but OUTSIDE all other sections of this file. That new line looks like this:
<uses-permission android:name="com.android.alarm.permission.RESERVE_TAXI_RESERVATION" />
The updated file should therefore look like this, with comments:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.android.intentspractise">
<!--The line below has been changed to give this app permission to reserve a taxi
N.B. The permission line HAS to be put here, inside the manifest section but outside the other
sections -->
<uses-permission android:name="com.android.alarm.permission.RESERVE_TAXI_RESERVATION" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
This should remove all of the red text and make your intent ready to use.
However, there is one more setback. Intents require your phone to have installed on it an app that can handle the intent. Unfortunately, I have been unable to find a Taxi app that can handle this intent. I have tried Uber andUudacity mentor @asheshb tried out some other taxi apps for me as part of all the wonderful help he offered me. To double check this I had to add an “else” statement with a line of code to make a Toast pop up:
Toast.makeText(this, "No App Available", Toast.LENGTH_SHORT).show();
This Toast only appears if the code successfully runs but there is no app able to handle the intent.
If anyone finds an app that can handle this intent please post the name of it in a reply below.
Please feel free to question, correct or further explain anything I have covered here. I am by no means an expert on this topic and would love anything that will help me learn more.
Links:
Comments