Android - Creating Intents: Camera
- Dan Thompson
- Feb 12, 2018
- 3 min read
Hello all,
I really enjoyed the Udacity Android Basics course by Google lesson Practice with Intents so I am taking some time to fully explore each of the intents in the Android Common Intents documentation. The one I have most recently looked at is Camera.
First I just created a simple button to activate the intent which looks like this:

And the code in activity_main.xml looks like this:
<Button
android:id="@+id/photo"
android:layout_width="@dimen/button_width"
android:layout_height="@dimen/button_height"
android:layout_gravity="center"
android:layout_margin="@dimen/margin"
android:onClick="takePhoto" android:text="Camera"/>
Then I created an activity in MainActivity.java to call the intent when the button is clicked. To launch a camera app all you need to do is include this code.
static final int REQUEST_IMAGE_CAPTURE = 1;
public void takePhoto(View view) {
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE);
}
}
Notice that the line: static final int REQUEST_IMAGE_CAPTURE = 1; is added above the intent and that this variable is then used in this line startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE); at the end of the intent. This variable is important when doing startActivityForResult because with these intents something is expected to be returned to your app and it needs a unique id number. Because it can only be a number it is helpful to turn it into a static variable so you can give it a more meaningful name.
In order to access and use the photo data from your camera, you need to write another block of code, shown below with comments:
/**
* This method takes the image your camera has taken and puts it into an ImageView
*/
@Override
// requestCode = REQUEST_IMAGE_CAPTURE
// resultCode lets you know whether or not the user took a photo
// data = the image the camera took
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// This line ensures that the user did, in fact take a photo before progressing
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
//This line gets the photo data
Bundle extras = data.getExtras();
//This line stores the photo data as a Bitmap variable
Bitmap imageBitmap = (Bitmap) extras.get("data");
// This line sends the photo data to an ImageView (with id mImageView) so it can be seen
mImageView.setImageBitmap(imageBitmap);
}
}
This needs you to do two more things before the image can be seen on your app screen. First you need to create an empty ImageView for the photo to be sent to, which should look like this:
<ImageView
android:id="@+id/mImageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Notice that the id for this ImageView @+id/mImageView is the same as the one used in the section of code above mImageView.setImageBitmap(imageBitmap);. You also need to add a line of code to the onCreate method and add a new Global variable. This will make the top of your Java code look like this:
private ImageView mImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mImageView = (ImageView) findViewById(R.id.mImageView);
}
The key changes that have been made here are the additions of the lines mImageView = (ImageView) findViewById(R.id.mImageView); inside the onCreate method and this line private ImageView mImageView; outside of the onCreate method. These two lines get the empty ImageView mImageView from the the activity_main.xml file then turns it into a global variable so that the onActivityResult method can use it to put he photo in.
All of this should allow you to use the Camera intent in your app and save a thumbnail of it on your app screen. At the end your screen should look something like this:
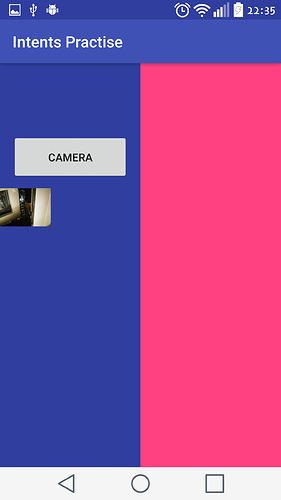
There is also a way to save and use the full image, not just a thumbnail and save in a particular place on your phone. There is also a way to use your phone to record video for your app. I hope to cover these and other Intents in other articles. If you are interested in this or get stuck I strongly suggest you watch the linked YouTube video as it helped me a lot!
Links:
Recent Posts
See AllI find that adding little notes to myself in my code is very helpful sometimes. It means that I can write pseudocode directly into...
Comments