Android - Creating Intents: Maps
- Daniel Thompson
- Jan 29, 2018
- 2 min read
Hello all,
I really enjoyed the Udacity - Android Basics lesson Practice with Intents so I am taking some time to fully explore each of the intents in the Android Common Intents documentation. The one I have most recently looked at is Maps.
First I just created a simple button to activate the intent. The app looks like this at first:
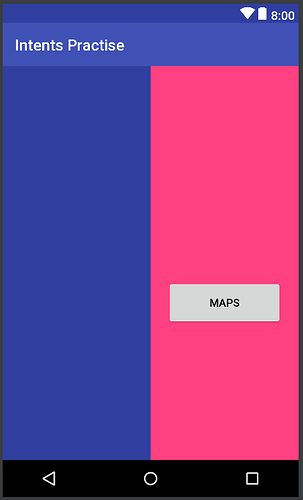
And the code in activity_main.xml looks like this:
<Button
android:id="@+id/showMaps"
android:layout_width="@dimen/button_width"
android:layout_height="@dimen/button_height"
android:layout_gravity="center"
android:layout_margin="@dimen/margin"
android:onClick="showMap"
android:text="Maps"/>
Then I created an activity in MainActivity.java to call the intent when the button is clicked. To launch a maps app all you need to do is include this code.
public void showMap(View view) {
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("geo:51.510357, -0.116773"));
if (intent.resolveActivity(getPackageManager()) != null) {
startActivity(intent);
}
}
Notice that the line: intent.setData(Uri.parse("geo:51.510357,-0.116773")); has replaced the example line from the Common Intents Map Guide intent.setData(geoLocation);. The word “geoLocation” is just some holder text in the guide you actually need to put in something like my example.
Let’s break down this line further. “intent” refers to the new ACTION_VIEW intent we just created on the line above. “.setData” means that we are about to add a data object to that intent, the data object is what follows within the paren. “Uri.parse” creates a new uri object out of a properly formatted string. The string in this case is “geo:51.510357,-0.116773”. This string is the geo-location of the centre of London near Big Ben. For note, both the signed degrees format and the DMS + compass directions format (which would be more like: N 51° 30’ 2’’ W 0° 7’ 28’’) seem to be accepted for geolocation.
I encourage you to explore what other geo-locations you can find on maps by either googling the latitude and longitude of your favourite places or using this website.
Next you can add a zoom to your maps view by adding “?z=” and then a number from 1-23 at the end of the geo-location. 1 is a view of the whole earth centered on the geo-location you put in and 23 is a close up on a portion of the road, etc. your geo-location has pinpointed. It seems to default at about zoom-level 15.
E.g. geo:51.510357,-0.116773?z=1:
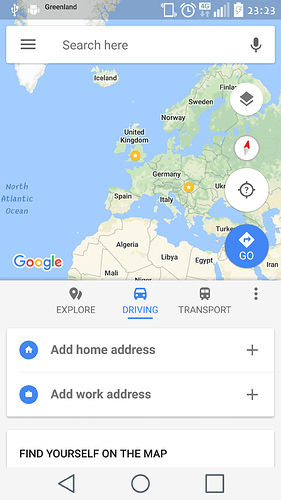
geo:51.510357,-0.116773?z=23
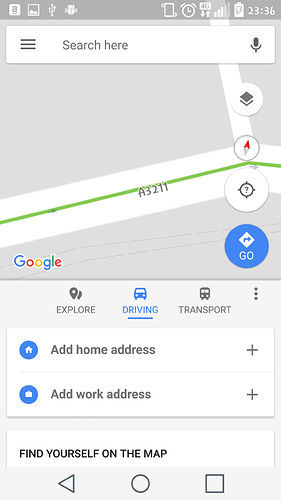
You can also add a note to your search by changing the line to this:
intent.setData(Uri.parse("geo:?q=51.510357, -0.116773(Treasure)"));
In this case the label given is “Treasure”. See the example:
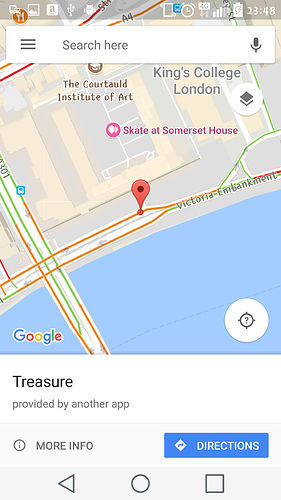
Finally, you can search for a location in the world by typing it into the geolocation like in this example:
intent.setData(Uri.parse( "geo:0,0?q=Big%20Ben%2C+UK"));
This line comes out in a google maps search as Big Ben, UK. Notice that “%20” and “+” have been used for spaces and “%2C” has been used for a comma. This is percent encoding and is required to make a Uri work. Click on percent encoding for a full list of acceptable characters in Uri’s and those that aren’t with the correct percent encoding. This final geolocation Uri actually got me closest to my target of Big Ben:
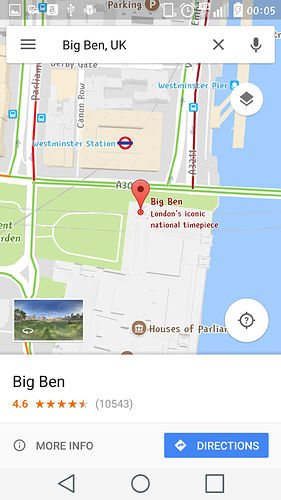
Please feel free to question, correct or further explain anything I have covered here. I am by no means an expert on this topic and would love anything that will help me learn more.
Links:
https://stackoverflow.com/questions/17467776/what-is-uri-parse-in-android
Recent Posts
See AllI find that adding little notes to myself in my code is very helpful sometimes. It means that I can write pseudocode directly into...
Komentáře