Android - Changing from Portrait to Landscape view
- Daniel Thompson
- Jan 25, 2018
- 4 min read
Hello all,
Recently, I have been tackling coding my app so that it does what I want when the screen is rotated from portrait to landscape view. This is something that I am finding a number of people are suggesting students do to improve their submissions for Project 2: Score Keeper so I thought I would share what I have learnt here in case it helps others.
To demonstrate the key points I have created a simple ScoreKeeper App in Android Studio. So far the main activity looks like this:

The Java file looks like this:
package com.example.android.rotatescreendemo;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle; import android.view.View;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
int teamAPoints = 0;
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void addOnePoint(View v) {
teamAPoints = teamAPoints + 1;
displayForA(teamAPoints);
}
public void displayForA(int point) {
TextView pointView = findViewById(R.id.team_a_score);
pointView.setText(String.valueOf(point));
}
}
Also my values folders look like this:
colors.xml:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
<color name="green">#008000</color>
<color name="red_color">#FF0000</color>
</resources>
strings.xml:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">Rotate Screen Demo</string>
<string name="team_name">Team A</string>
<string name="score">0</string>
<string name="plus_symbol">+</string>
<string name="minus_symbol">-</string>
</resources>
dimens.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="text_size">40sp</dimen>
<dimen name="button_size">40dp</dimen>
<dimen name="margin_size">10dp</dimen>
</resources>
Now we have our code we need to put it in landscape! Now, on your phone, this may be obvious. You just turn it sideways. Honestly, I had not even thought of doing that to test my own apps until I got to this part of the course! But how do you get a landscape preview in Android Studio? Focus on the preview pane of Android Studio:
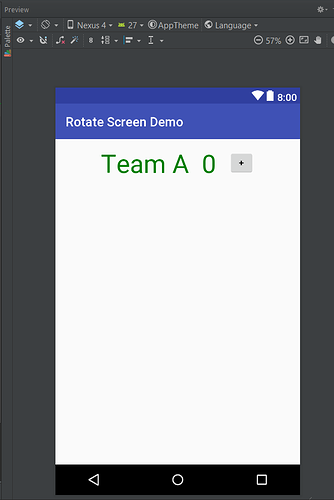
Look up at the top left corner for this button:

Click on it and it will open a new menu. First use this menu to switch your preview landscape using either “Switch to Landscape” or “Landscape”:
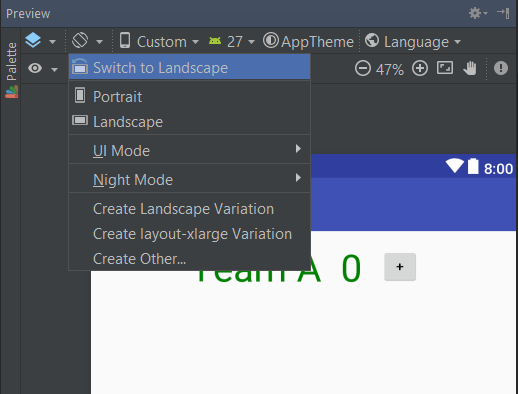
Pretty cool, huh? But the app looks the same whichever way up it is - except for maybe too much or too little space in landscape mode because you didn’t design your app for that view yet. We’re about to fix that. See the other option in that pop-up menu that says “Create Landscape Variation”? Click it.
Now you can see a new tab at the top of your middle, coding pane that says “land\activity_main.xml”
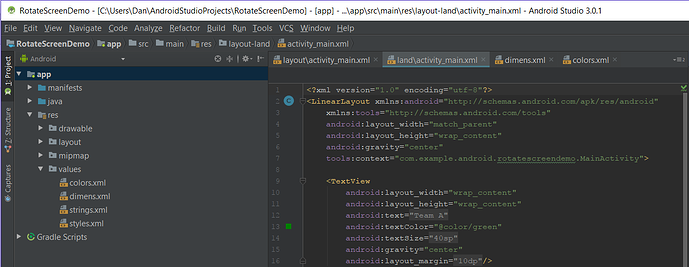
You can switch back to your main or portrait XML document by clicking on the “layout\activity_main.xml” tab next to the other tab. Can’t see it? Don’t worry, click on this button:

(yours may have a different number than 2 next to it, that’s fine) and click on "layout\activity_main.xml from that drop-down menu. You can also see the folder this new file is in if you double click on: "Layout Land" on this horizontal menu near the top of Android Studio:

This will change the right-column of Android Studio into Project mode with the layout-land folder open and a file called “activity_main.xml” visible inside it.
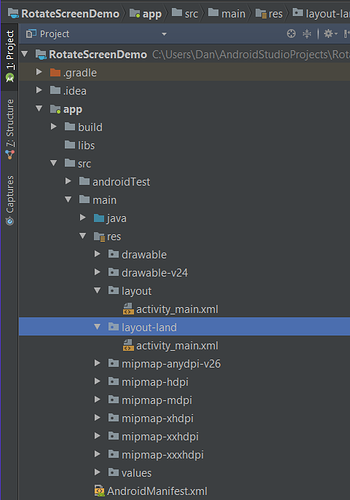
You will notice that just above the “layout-land” folder is another folder called just “layout” and inside that is another file also called “activity_main.xml”. It is absolutely fine that these both have the same name. The two folders separates these documents into one that controls the portrait view, just “layout”, and one that controls the landscape view, “layout-land”.
By the way, if you don’t like the right column of Android Studio to remain in Project mode you can click on where it says Project at the top of this column and you will get another drop-down menu. Select “Android” to go back to the view we are used to. I prefer the Android view but you cannot see the “layout-land” folder while in it.
Anyway, let’s return to the land/activity_main.xml file itself to actually change how our app appears in landscape mode. Now, you may have noticed that the code in this file is currently identical to the code in the activity_main.xml file. If you haven’t noticed take a second to flick again between the two tabs to check.
Back? Didn’t do it? What do I care? I’m a fellow student, not a teacher.
The reason why both these files are currently identical is because when we clicked on “Create Landscape Variation” a few minutes ago it just automatically copied the contents of activity_main.xml into this new folder. To change how your app looks in landscape mode we need to change the code in land/activity_main.xml. You can change yours in any way you like: adding or changing Layouts; making Views bigger or smaller; moving views about; whatever you need to do to make it look as good in Landscape mode as it is in portrait. To make this demonstration simple, and to stop it getting much longer, I am just going to change the text color from green to red. To do this, all I had to do was change:
android:textColor="@color/green"
In both of the TextViews in land/activity_main.xml ONLY to:
android:textColor="@color/red_color"
I could do this because I had already prepared this in the colors.xml values folder otherwise I could have hard-coded this new color using:
android:textColor="#FF0000"
So now when my phone is portrait my app still looks like this:
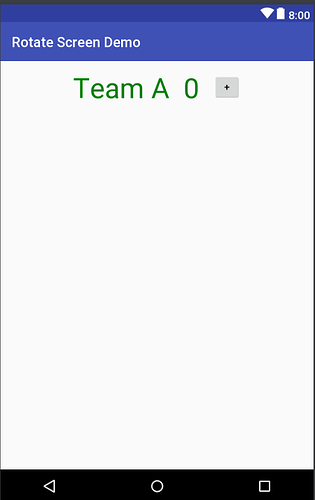
but in landscape mode it now looks like this:
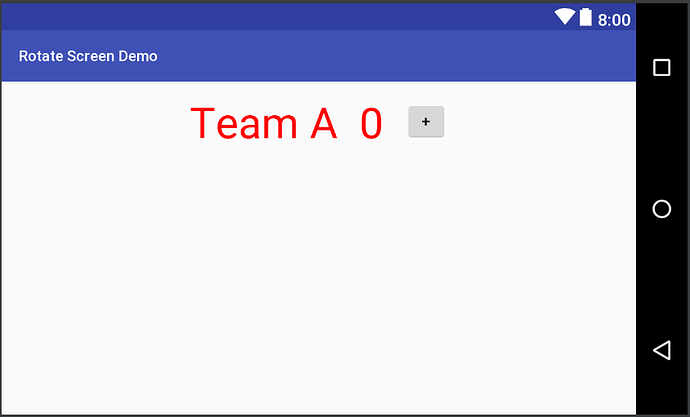
I hope that this has helped anyone who is struggling to get their heads around this topic. I know it took me a long time, a lot of reading and support from awesome moderators on the Udacity Discussion forums and Slack channels to get me there.
Thanks for reading and if it helps share it with others who are struggling.
Links:
https://programthat.wordpress.com/2013/04/30/how-to-set-up-a-dimensions-xml-file/
https://stackoverflow.com/questions/17146929/how-to-create-new-res-folder-in-android-studio http://tekeye.uk/android/examples/ui/android-portrait-landscape-screens2
Recent Posts
See AllI find that adding little notes to myself in my code is very helpful sometimes. It means that I can write pseudocode directly into...
Comments